Visual Basic is an event driven programming language that can run on Microsoft windows environment. It is a successor of BASIC language and is visual in nature. Visual Basic was developed in early 1990s by Microsoft Corporation.Visual Basic is a visual programming language which involves a lot of graphics rather than writing numerous lines of code to illustrate the appearance, functionality, etc. of the applications interface. It is a WYSIWYG (What you see is what you get) editor.
Visual Basic provides acommon Programming platform across all MS Office applications.
Events
An event refers to the occurance of an activity. Eg: When you click a command button a set of statments are executed. Comman Button is the object and click is the event(action).
Event Procedure
An event procedure is a procedure containing code that is executed when a event occurs.
Double clicking an object will take you to the default event of an object. Here on double clicking the button you are taken to the click event.
Eg :
Private Sub btnDisplay_Click()
Print "Welcome to Visual Basic"
Print "Shalvin"
End Sub
Here the two print statements are contents of the procedure which is related to the click event.
The output is shown in the figure below.
Properties
Properties are the attributes or characteristics of an object.Eg: Name, Caption, Font, etc. are the properties of a Label. Properties can be set either using the Properties Windows or by writing code.
Visual Basic 6 Editions
Learning EditionFor beginners. It is also known as Standard Edition. It includes all intrinsic controls.
Professional EditionIncludes all the features of Learining Edition, in addition it has activex Controls and Visual Data Tools.
Enterprise EditionThis edition is used to create distributed application in a team setting.
Enabled and Visible Properties
Enabled Property
Used to set a value that determines if the label canl respond to the events that are generated by the user. If the enabled Property is false that particular control will be inactive.
Visible Property
Determines whether the control will be visible or invisible on the screen.
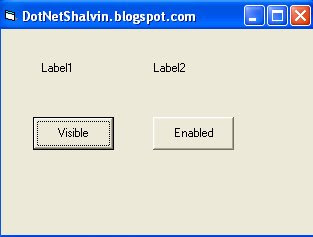
Private Sub btnEnabled_Click()
Label2.Enabled = True
End Sub
Private Sub cmdVisible_Click()
Label1.Visible = False
End Sub
Result
Variables and Data Types
Variable
Variables are named storage locations whose values can be manipulated during execution of the programme.
A variable has a name ane data type.
Data Type
Data Type refers to type of data and associated operations to identify a particular data.
Data Types can be broadly classified into :
1. Numeric Types
2. Non Numeric Types
Numeric Types
They consist of numbers.
Non numeric types
It deals with a character or set of characters, date or boolean value (true/false).
Numeric Data Types
Byte - 0 to 255
Integer - -32,767 to 32,767
long - 4 bytes
Single (decimal numbers) - 4 bytes
Double (decimal numbers) - 8 bytes
Non Numeric Data Types
String - Single character or set of characters
Date
Boolean - True of false
Variant - If data type is mentioned while declaring a variable, it is considered as variant.
Dim statment is used to define a variable.
Suppose you want to define a string memory named strName of type string.
Dim strName as string
Operators
Operators are symbols or words that trigger an action on some data.
Types of operators
Arithmetic Operators
Concatenation Operators
Comparison Operators
Logical Operators
Arithmetic Operators
Arithmetic Operators are the operators based on mathematical calculations.
+ Addition
- Sutraction
* Multiplication
/ Division
mod Reainder after division
^ Exponent
Concatenation Operators (&, +)
Concatenation operators are used to join two or three variables. If it is string data type, it will be concatenate both the values.
If it is integer data type both the values will be added.
Comparison Operators
Comparison operators are used to compare two values.
> Greater than
>= Greater than or equal to
< Less than
<= Less than or equal to
= Assignment operator and equality checking operator
<> Not equal to
Logical Operators
Logical operators are used to compare two conditions.
AND
XOR
OR
LIKE NOT
Menus
Menus are a convenient and consistent way to group commands so that they become readily and easily available to the users.
To create a menu,
Tols -> Menu Editor or clicking the Menu Editor icon in the Standard Toolbar as shown in the figures below.
Fundamentals of menu
Menu Bar
Menu Bar is a horizontal bar containing different menu options. Each menu option has sub menus.
Pull Down Menu
When you click an option on a menu bar you will get submenus under that, those menus are called pull down menus (vertical menu).
Menu Item
Each option on a menu bar is called menu item.
Sub Menu
A menu attached to a menu item is called a sub menu.
Pop up Menu
A floating menu that is displayed over a form or a control independent of the menu bar. It is also called Context menu.
Separator Bar
A bar on a menu that divides menu items into logical groups on a menu.
Shortcut Key
Key or a key combination used for invoking the command associated with a menu item.
Assigning Access Key
Access keys allow the user to open a menu by pressing the alt key and typing a designated letter. To assign an access key, select the menu item and set the caption as & immediately infront of the letter which is the starting letter of the corresponding menu item.
Creating a Calculator
Dim i, j, res As Integer
Private Sub btnAdd_Click()
Assign
res = i + j
lblResult.Caption = res
End Sub
Private Sub Assign()
i = Val(txtInt1.Text)
j = Val(txtInt2.Text)
End Sub
Private Sub btnDivide_Click()
On Error GoTo Err
Assign
res = i / j
lblResult.Caption = res
Err:
If Err.Number = 11 Then
MsgBox "Cannot divide a number with 0"
txtInt2.Text = ""
txtInt2.SetFocus
End If
If Err.Number = 6 Then
MsgBox "Input not in correct format"
End If
End Sub
Database Connectivity with ADO
ActiveX Data Object (ADO) is the preferred database connectivity option for VB 6. Inorder to work with ADO you have to set a reference to Microsoft ActiveX DataObject 2.x Library by going to Project, Add Reference Dialog.
Dim cnn As ADODB.Connection
Dim rs As ADODB.Recordset
Private Sub Form_Load()
Set cnn = New ADODB.Connection
cnn.Open "Provider=SQLOLEDB.1;Integrated Security=SSPI;Initial Catalog=Northwind"
Set rs = cnn.Execute("select * from Categories")
While Not rs.EOF
List1.AddItem (rs("CategoryName"))
rs.MoveNext
Wend
End Sub
Related Blog
VB.Net : The Console Way
No comments:
Post a Comment