You can use the ServiceController class (System.ServiceProcess) to connect to and control the behavior of existing services.
Enumerating services
using System.ServiceProcess;
private void Form1_Load(object sender, EventArgs e)
{
foreach (ServiceController s in ServiceController.GetServices())
listBox1.Items.Add(s.DisplayName );
}
You can then use the class to start, stop, and otherwise manipulate the service.
Here I am using ServiceController class to start and stop Sql Server 2000 Service.
using System.ServiceProcess;
ServiceController sc = new ServiceController();
private void Form1_Load(object sender, EventArgs e)
{
sc.ServiceName = "MSSqlServer";
}
private void btnStart_Click(object sender, EventArgs e)
{
if (sc.Status == ServiceControllerStatus.Stopped sc.Status == ServiceControllerStatus.Paused )
{
sc.Start();
MessageBox.Show("Service started");
}
else
MessageBox.Show("Service already started");
}
private void btnStop_Click(object sender, EventArgs e)
{
if (sc.Status == ServiceControllerStatus.Running )
{
sc.Stop();
MessageBox.Show("Service stopped");
}
else
MessageBox.Show("Service alredy in stopped state");
}
}
Monday, December 15, 2008
ServiceController Class for Managing a service
Friday, December 12, 2008
Isolated Storage (Code Snippets)
using System.IO;
using System.IO.IsolatedStorage;
private void button1_Click(object sender, EventArgs e)
{
this.Text = textBox1.Text;
IsolatedStorageFile f = IsolatedStorageFile.GetMachineStoreForDomain();
IsolatedStorageFileStream i = new IsolatedStorageFileStream("co.col", System.IO.FileMode.Create, f);
StreamWriter sw = new StreamWriter(i);
sw.Write(this.Text);
sw.Flush();
sw.Close();
}
private void Form1_Load(object sender, EventArgs e)
{
IsolatedStorageFile f1 = IsolatedStorageFile.GetMachineStoreForDomain();
IsolatedStorageFileStream i1 = new IsolatedStorageFileStream("co.col", FileMode.Open, f1);
StreamReader sr = new StreamReader(i1);
this.Text = sr.ReadToEnd();
sr.Close();
}
Courtesy : Saji P Babu, IndiaOptions, Kochi.
Related Blog
System.IO Namespace in .Net
Wednesday, December 10, 2008
Linq to Xml (Code Snippets)
Linq to Xml enables you to query an Xml document using the familiar LINQ syntax. Linq to Xml features and found within System.Xml.Linq namespace.
System.Xml.Linq introduces a series of objects such as XDocument, XElement and XAttributes which makes creating XML document a breeze.
XAttribute
private void btnXElement_Click(object sender, RoutedEventArgs e)
{
XElement xe = new XElement(new XElement("Shalvin",
new XElement("Students",
new XElement("Name", "Shalvin"))));
textBox1.Text = xe.ToString();
}
XElement
using System.Xml.Linq;
private void Form1_Load(object sender, EventArgs e)
{
XElement xml = new XElement("contacts",
new XElement("contact",
new XAttribute("contactId", "2"),
new XElement("firstName", "Shalvin"),
new XElement("lastName", "PD")
),
new XElement("contact",
new XAttribute("contactId", "3"),
new XElement("firstName", "Praseed"),
new XElement("lastName", "Pai")
)
);
textBox1.Text = xml.ToString();
}
XDocument
using System.Xml.Linq;
protected void Page_Load(object sender, EventArgs e)
{
XDocument xd = new XDocument();
XElement contacts =
new XElement("contacts",
new XElement("contact",
new XElement("name", "Shalvin"),
new XElement("Location", "Kochi")
),
new XElement("contact",
new XElement("name", "Viju"),
new XElement("Location", "Trichur")
)
);
xd.Add(contacts);
xd.Save("c:\\Shalvin.xml");
System.Diagnostics.Process.Start("iexplore", "c:\\Shalvin.xml");
}
Output
<?xml version="1.0" encoding="utf-8"?>
<contacts>
<contact contactId="2">
<firstName>Shalvin</firstName>
<lastName>P D</lastName>
</contact>
<contact contactId="3">
<firstName>Praseed</firstName>
<lastName>Pai</lastName>
</contact>
</contacts>
XDocument Parse
private void button3_Click(object sender, RoutedEventArgs e)
{
XDocument xd = XDocument.Parse(@"<contacts>
<contact contactId='2'>
<firstName>Shalvin</firstName>
<lastName>P D</lastName>
</contact>
<contact contactId='3'>
<firstName>Praseed</firstName>
<lastName>Pai</lastName>
</contact>
</contacts>");
textBox1.Text = xd.ToString();
}
Querying an Xml Document
using System.Xml.Linq;
private void Form1_Load(object sender, EventArgs e){
XDocument xmlFile = XDocument.Load("c:\\Shalvin.xml");
var query = from c in xmlFile.Elements("contacts").Elements("contact").Elements("name")
select c;
foreach (XElement nam in query)
{
listBox1.Items.Add(nam);
}
}
Related Blogs
Linq to Objects
Linq to Sql
.Net Working with Xml
Monday, December 8, 2008
Linq to Objects
LINQ (Language Integrated Query) is a Microsoft .NET framework component that adds native data querying capabilities to C# and Visual Basic .NET (VB.NET) programming languages.
It allows developers to write database-like queries against various data sources including in-memory objects, SQL databases, and XML data sources.
LINQ enables developers to perform queries in a more concise, readable and expressive way, compared to traditional loops and conditional statements.
This blog assumes that you are know Type Inference in C#, Generics and Collection Initializer.
private void Form1_Load(object sender, EventArgs e)
{
List<string> interests = new List<string>{"Music", "Singing", "Swimming"};
var result = from w in interests
where w.Contains("ing")
select w;
foreach (string s in result)
listBox1.Items.Add(s);
}
Lambda
protected void btnLambda_Click(object sender, EventArgs e)
{
List<string> interests = new List<string>{"Music", "Singing", "Swimming"};
var result = interests.Where(p => p.Contains("ing"));
foreach (string s in result)
ListBox1.Items.Add(s);
}
The Linq query retrives all values form Generic List that ends with "ing". You can use Linq with any objects that implements IEnumerable or Generic IEnumerable interface.
StartsWith
private void btnStartsWith_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = from p in interests
where p.StartsWith("M")
select p;
foreach (string s in ms)
listBox1.Items.Add(s);
}
EndsWith
private void btnEndWith_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = from p in interests
where p.EndsWith("g")
select p;
foreach (string s in ms)
listBox1.Items.Add(s);
}
Equals
private void btnEquals_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = from p in interests
where p.Equals("Music")
select p;
foreach (string s in ms)
listBox1.Items.Add(s);
}
First
First can be used to extract only the first item in the collection.
private void btnFirst_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = (from p in interests
select p).First();
listBox1.Items.Add(ms);
}
Last
private void btnLast_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = (from p in interests
select p).Last();
listBox1.Items.Add(ms);
}
Count
Using the Count operator I am taking a counts of items inside the Generic List. I will get a value 3.
private void btnCount_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = (from p in interests
select p).Count();
listBox1.Items.Add(ms);
}
Count of EndsWith
Here I am taking a count of items ending with "ing". I will get an output of 2 ie. the count of "Swimming" and "Singing".
private void btnCountEndWithIng_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = (from p in interests
where p.EndsWith("ing")
select p).Count();
listBox1.Items.Add(ms);
}
Count Equals
private void btnCountEquals_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var ms = (from p in interests
where p.Equals("Music")
select p).Count();
listBox1.Items.Add(ms);
}
Linq and Generic List of Integer
Having seen working with Generic List of string let's turn out attention to Generic List of Inteters.
List<int> gliMarks = new List<int> { 98, 56, 88, 79, 96 };
GreaterThan Operator
private void btnShowGreaterThan90_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var G90 = from p in gliMarks
where p > 90
select p;
listBox1.ItemsSource = G90;
}
Sum
private void btnSum_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var vSum = (from p in gliMarks
select p).Sum();
listBox1.Items.Add(vSum);
}
Average
private void btnAverage_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var Avg = (from p in gliMarks
select p).Average();
listBox1.Items.Add(Avg);
}
Max
private void btnMaximum_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var Maxi = (from p in gliMarks
select p).Max();
listBox1.Items.Add(Maxi);
}
Or
private void btnSOr_Click(object sender, RoutedEventArgs e)
{
listBox1.Items.Clear();
var vOr = from p in gliMarks
where p== 98 | p == 88
select p;
listBox1.ItemsSource = G90;
}
Linq on Collection of Objects
class Session
{
public string Speaker { get; set; }
public string SessionName { get; set; }
}
List<Session> glSessions = new List<Session>{
new Session{Speaker = "Shalvin P D", SessionName= "Entity Framework 4"},
new Session{Speaker="Praseed Pai", SessionName="Powershell"}};
var G = from p in glSessions
where p.Speaker == "Shalvin P D"
select new { p.Speaker, p.SessionName };
dataGrid1.ItemsSource = G.ToList();
}
Type Inference in C# (Implicitly Typed Local Variable)
Type inference is a new feature in C# 3.0. With Type Inference you allow compiler to determine the type of a local variable at compile time.
private void Form1_Load(object sender, EventArgs e)
{
var strName = "Shalvin";
MessageBox.Show(strName.GetType().ToString());
var intPoints = 67;
MessageBox.Show(intPoints.GetType().ToString());
var dblBalance = 110.50;
MessageBox.Show(dblBalance.GetType().ToString());
var cBool = true;
Console.WriteLine(cBool.GetType());
var cDate = DateTime.Now;
Console.WriteLine(cDate.GetType());
}
The first batch of statements creates a memory variable of type System.String.
The Second batch of statements (intPoints) creates a memory variable of type System.Int32.
The third batch of statments (dblBalance) creates a memory variable of type System.Double.
The statement var cBool = true; creates a memory variable of type System.Boolen.
The state var vDate = DateTime.Now; creates a memory variable of type System.DateTime.
Related Blog
Generics and Collection Initializer in .Net
Friday, November 28, 2008
Currency Conversion Web Service with Asp .Net
I stumbled upon a excellent Currency Converter Web Service http://www.webservicex.net/CurrencyConvertor.asmx.
So thought of blogging on consuming a web service with Asp .Net.
Start a new Asp .Net project and Add web reference to the above mentioned web service.
Here is the code for invoking the ConversionRate method.
CurrencyConvertor.CurrencyConvertor cc = new CurrencyConvertor.CurrencyConvertor();
double dblConv;
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnUSDollar_Click(object sender, EventArgs e)
{
dblConv = cc.ConversionRate(CurrencyConvertor.Currency.USD,
CurrencyConvertor.Currency.INR);
lblConversion.Text = dblConv.ToString();
}
A few useful web services
http://webservices.oorsprong.org/websamples.countryinfo/CountryInfoService.wso
Related Blog
.Net Remoting Walkthrough
Thursday, November 27, 2008
AJAX .Net VIII : PasswordStrength Extender
PasswordStrength extender provides immediate feedback to the user about the strength of a password based on the site's Password Strength policy.
Add an Ajax Web Form or a classical Asp .Net Page and add a ScriptManager instead.
PasswordStrength extends a TextBox.
Add a TextBox and set the TextMode to Password. Add a PasswordStrength extender and set its TargetId to the TextBox.
HelpStatusLabelId Property
If you want extra information on the status of Password Strength you can use HelpStatusLabelId property.
Place a Label and set the HelpStatusLabelId Property of PasswordStrength extender control to the Label.
MinimumSymbolCharacters Property
The above example expects the user to enter 10 characters. But in many cases the site expects you to provide additional special characters, numeric characters and the like to make a strong password for this you can use properties like MinimumSymbolCharacters, MinimumLowerCaseCharacters, MinimumUpperCaseCharacters and MinimumNumericCharacters properties of PasswordStrength extender control.
In this example I am setting MinimumSymbolCharacters of PasswordStrength extender control to 1. As shown in the figure the user is expected to enter a special character to make it unbreakable.
PasswordStrength BarIndicator
We exploring TextIndicator option of PasswordStrength. It is possible to have BarIndicator for a PasswordStrength extender control.
You should have a CSS class for enabling BarIndicator as shown in the figure below.
Now you can specify the CSS class in BarBorderCssClass property.
cc1:PasswordStrength ID="PasswordStrength1" runat="server"
StrengthIndicatorType="BarIndicator"
TargetControlID="txtPassword"
BarIndicatorCssClass = ""
BarBorderCssClass="BarBorder"
The user is expected to provide BarIndicatorCSSClass property also. To make things simple I am omitting the value.
Wednesday, November 26, 2008
Themes in Asp .Net
Themes can be used to have a consistent look for User interface elements in Asp .Net.
Add a skin by selecting Skin from Add New Item dialog, give a good name, say Blue. This will create a folder called Blue inside App_Themes special folder.
A Skin File is used to set properties for Controls.
Select the newly created Skin file. Inside that you can specify the control properties as shown in the figure below.
Here I have specified the Text Box to have light Yellow BackColor and Button to have Light Blue BackColor.
Now go back to the Asp .Net page and create the visual interface. Set the StyleSheetTheme property of document to the newly created SkinFile.
Now the controls will inherit the properties you have specified in the skin file.
Setting site wide theme in web.config
Instead of setting StyleSheetTheme property for individual forms go to web.config and identify the Page tag and set theme attribute to the newly created skin file.
Instead of directly tweaking the properties by going to properties windows, I used to place a control in the Asp .Net page set appropriate properties then navigate to the source and cut the control's tag and paste it in the skin file. While doing so ensure that you remove the id attribute.
Adding CSS File to Themes
It is possible to have a CSS file in themes folder. Select the themes folder and select Add New Item from WebSite menu. All files related to the current selection ie. themes will appear. Select Style Sheet.
A style sheet can be used to specify html styles.
Here I am setting the background color of the form.
Related Blog
ASP.Net Master Page for Consistant look and feel for websites
Friday, November 21, 2008
Silverlight 3, WPF Positioning with ZIndex
You can use ZIndex property to change the order in which controls are rendered on a canvas.
The control with highest ZIndex value will be shown at the very top. Here Blue rectangle is having ZIndex 3, Yellow having ZIndex 2, so Blue rectanle will appear at the very top, followed by Yellow rectange and Cyan rectangle.
Programatically changing the ZIndex
private void Button_Click(object sender, RoutedEventArgs e)
{
Canvas.SetZIndex(rectCyan, 4);
}
Monday, October 27, 2008
Filling Windows Forms ListView with DataTable
If your are not familiar with List visit my blog Windows Forms ListView.
DataTable dt = new DataTable("Shalvin");
DataRow dr;
DataColumn dc;
private void Form1_Load(object sender, EventArgs e)
{
listView1.View = View.Details;
listView1.Columns.Add("Blog", 170);
listView1.Columns.Add("Author", 170);
dc = new DataColumn("Blog");
dt.Columns.Add(dc);
dc = new DataColumn("Author");
dt.Columns.Add(dc);
dr= dt.NewRow();
dr["Blog"] = "http://weblogs.asp.net/Scottgu/";
dr["Author"] = "Scott Guthrie";
dt.Rows.Add(dr);
dr = dt.NewRow();
dr["Blog"] = "DotNetShalvin.blogspot.com";
dr["Author"] = "Shalvin";
dt.Rows.Add(dr);
listView1.Items.Clear();
To make things simple I am creating a DataTable and DataRows by code .Afterward I am iterating through the DataRows collection and adding it into ListView's SubItems collection.
private void Form1_Load(object sender, EventArgs e)
{
..
..
ListViewItem lvbl = new ListViewItem("Add new record");
listView1.Items.Add(lvbl);
}
private void listView1_KeyPress(object sender, KeyPressEventArgs e)
{
if (listView1.FocusedItem.Text.ToString() == "Add new record")
MessageBox.Show("Add new record");
}
VB .Net
Dim dt As New DataTable("Shalvin")
Dim dr As DataRow
Dim dc As DataColumn
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
ListView1.View = View.Details
ListView1.Columns.Add("Blog", 170)
ListView1.Columns.Add("Author", 170)
dc = New DataColumn("Blog")
dt.Columns.Add(dc)
dc = New DataColumn("Author")
dt.Columns.Add(dc)
dr = dt.NewRow()
dr("Blog") = "http://weblogs.asp.net/Scottgu/"
dr("Author") = "Scott Guthrie"
dt.Rows.Add(dr)
dr = dt.NewRow()
dr("Blog") = "DotNetShalvin.blogspot.com"
dr("Author") = "Shalvin"
dt.Rows.Add(dr)
For index As Integer = 0 To dt.Rows.Count - 1
Dim dr As DataRow = dt.Rows(index)
Dim lvi As New ListViewItem(dr("Blog").ToString())
ListView1.Items.Add(lvi)
lvi.SubItems.Add(dr("Author"))
Next
Related Blog :
DataTable DataColumn and DataRow (Asp .Net)
DataGridView - Extracting the Contents of Currently Selected Row
FileSystemWatcher Component and Class in WPF and Windows Forms
FileSystemWatcher Component (Class) can be used to monitor file system directories for changes. FileSystemWatcher Class in WPF FileSystemWatcher Class in found in System.IO namespace using System.IO is not added by default in WPF. using System.IO; private void Window_Loaded(object sender, RoutedEventArgs e) { FileSystemWatcher fsw = new FileSystemWatcher(); fsw.Path = @"c:\"; fsw.IncludeSubdirectories = true; fsw.EnableRaisingEvents = true; fsw.Created +=new FileSystemEventHandler(fsw_Created); } private void fsw_Created(object sender, FileSystemEventArgs e) { MessageBox.Show(e.FullPath + " created"); } FileSystemWatcher Component in Windows Forms Add a FileSystemWatcher to the form. Since it is a component it will appear in the component tray. Support if you want to monitor c: and its sub directories for changes, then set the Path property to C:, IncludeSubdirectories to True and EnableRaisingEvents to true. Now you can write event handlers for the FileSystemWatcher Component as follows: private void fileSystemWatcher1_Created(object sender, System.IO.FileSystemEventArgs e) { MessageBox.Show(e.FullPath + " created"); } private void fileSystemWatcher1_Deleted(object sender, System.IO.FileSystemEventArgs e) { MessageBox.Show(e.FullPath + " deleted"); } A better approach would be writing to an event log or a text file. Related blog: DataTable DataColumn and DataRow (Asp.Net)
Sunday, October 26, 2008
LINQ to Sql
I am going to demonstrate LINQ to Sql by writing code.
Support you have a table called Categories in Shalvin database you need to first create a Entity. An Entity is a class that represents a Table or a view in the database.
Create a class as follows:
Set a reference to Data.Linq.
using System.Data.Linq.Mapping;
[Table]
public class Categories
{
[Column(IsPrimaryKey=true, IsDbGenerated=true)]
public int CategoryId { get; set; }
[Column]
public string CategoryName { get; set; }
[Column]
public string Description { get; set; }
}
Each property should have Column Atribute. I am also setting values of IsPrimaryKey and IsDbGenerated for CategoryId column. Editing is possible only if IsPrimaryKey is set to true. IsDbGenerated Indicated that it is an Identity column.
In the asp .net page :
using System.Data.Linq;
protected void Page_Load(object sender, EventArgs e)
{
string ConString = "Integrated Security=sspi;Initial Catalog=Shalvin";
DataContext db = new DataContext(ConString);
var tMovies = db.GetTable<categories>();
GridView1.DataSource = tMovies;
DataBind();
}Now suppose you are interested only in viewing the Categories table with CategoryName 'Confections'.
GridView1.DataSource = tMovies.Where(m => m.CategoryName.Contains("Beverages"));The DataContext is the source of all entities mapped over a database connection. It tracks changes that you made to all retrieved entities .
Linq to Sql with Visual Studio Tools
Having seen the internals lets turn our attention to the Visual Studio Support of Linq to Sql.
Here I have started an Empty Visual Studio Project and Added a Linq to Sql Classes from the Add New Item Dialog. I have renamed it AirLines.dbml
That's all I have to do and I am ready to do CRUD operation with the selected tables.
AirLinesDataContext context = new AirLinesDataContext();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
GridView1.DataSource = context.Locations;
DropDownList1.DataSource = context.Locations;
DropDownList1.DataTextField = "LocationName";
DropDownList1.DataValueField = "LocationId";
DataBind();
}
}
protected void DropDownList1_SelectedIndexChanged(object sender, EventArgs e)
{
Response.Write(DropDownList1.SelectedValue.ToString());
}
protected void btnInsertLocation_Click(object sender, EventArgs e)
{
var loc = new Location { LocationName = "London", Latitude =67 };
context.Locations.InsertOnSubmit(loc);
context.SubmitChanges();
}
Related Blogs
Linq to Objects
Linq to Xml (Code Snippets)
Wednesday, October 15, 2008
Microsoft Innovation Days Kochi
Learn for free about building reliable, high performance applications with a super-rich user interactivity using Microsoft technologies, such as the Visual Studio 2008, SQL Server 2008, Windows Server 2008, Silverlight 2 RC0 and Internet Explorer 8.
Date: November 6, 2008 (Thursday)
Venue: Taj Residency
Marine Drive, Shanmugham Road
Ernakulam, Cochin 682304
9 am Tea and Registration
9:45 AM Keynote - The magic of Software
Sneak peak into the host of technology innovations that Microsoft is working on - Windows 7, Live platform, Cloud services and many more , accompanied with uber-cool demos.
10:45 AM Next generation web experience with IE 8 and Silverlight
Learn how you can leverage some of the developer features in IE 8 to "light up" your web site and provide a compelling experience to your website users by rendering your data on Silverlight controls.
12:00 PM SQL Server – Optimize your Queries
Lunch Break (1.30 - 2.30)
2:30 PM Partnering with Microsoft
Learn about the various partnering opportunities with Microsoft that will support and enhance your development and marketing efforts.
3:00 PM AJAX - Get it right for your ASP.NET Applications
Find out how to AJAX Enabling your ASP.NET Applications in the right way and also explore some of the best practices to build a high performance web application. Finally, learn how the new version of ASP.NET improves AJAX enabled web development.
4:30 PM The developer's toolkit for building .Net applications.
Find out the host of tools you can use to analyze and identify performance bottlenecks in your .Net applications. Explores some of the in-built tools that ships with the .NET SDK such as ildasm, gacutil, ngen, fxcop etc and and features in Visual Studio. which will help you develop better, faster, more secure, error free NET applications.
Click here to register
Friday, October 10, 2008
Silverlight 3/Wpf : Adding Controls Dynamicallys
Lets see how to dynamically add controls and attaching event handler to the added control.
Here I am creating an object of button class and adding it into the Grid using the Add method of Grid's Children property.
private void btnAddControl_Click(object sender, RoutedEventArgs e)
{
Button btn = new Button();
btn.Click += new RoutedEventHandler(btn_Click);
btn.Content = "Shalvin";
btn.Width = 75;
btn.Height = 25;
Grid1.Children.Add(btn);
}
private void btn_Click(object sender, RoutedEventArgs e)
{
//MessageBox works only with WPF , Silverlight 3 and not with Silverlight 2
MessageBox.Show("ShalvinPD.blogspot.com");
}
Result
WPF Routed Event
A routed event is a type of event that can invoke handlers on multiple listeners in an element tree, rather than just on the object that raised the event.
For example if you want a single event handler for the buttons inside a Grid you can define a Routed event as Button.Click = "Grid_Click" inside the Grid as shown in the diagram.
private void Grid_Click(object sender, RoutedEventArgs e)
{
//MessageBox works only with WPF and not Silverlight 2
MessageBox.Show("ShalvinPD.blogspot.com");
}
Wednesday, October 8, 2008
WPF Templates
WPF introduces the concept of lookless controls.
Using Templates it is possible to change the appearance of a control. For examples it is possible for a ListBox to contain the Photos of employees instead of the usual List Items.
I am going to demonstrate creating a simple Template whereby a button will appear as a blue rectangle.
Silverlight Tags
<UserControl x:Class="ButtonTemplate.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<Button Height="50" Width="75">
<Button.Template>
<ControlTemplate>
<Rectangle Fill="LightBlue"/>
</ControlTemplate>
</Button.Template>
</Button>
</Grid>
</UserControl>
Result
As shown in the output the button can respond to click event as expected.
Now lets create an ellipse button.
Result
Monday, October 6, 2008
WPF Styles
Styles are equivalent to CSS in html. Lets see how to create Styles in WPF.Inside the Window Xaml tag add a Window.Resource section.In the Style tag's TagetType you can specify to what type of control you want the style to apply. Using Setters you can specity the Property and and its corresponding value.
Here is the result
In this example I am setting the Background, Width and Opacity properties for Button. All the buttons in the form will inherit these properties thereby rendering a consistant look and feel.
Wednesday, September 3, 2008
Asp .Net Validation Controls
RequiredFieldValidator
RequiredFieldValidator can be used to check whether a value is entered into a control.
Place a RequiredFieldValidator next to the control you want to check for the extistence of value.
Set the ControlToValidate Property to the Control you want to validate. Set an appropriate errror message using the ErrorMessage property. If you submit the form without entering the a value to the TextBox the use will receive an error message.
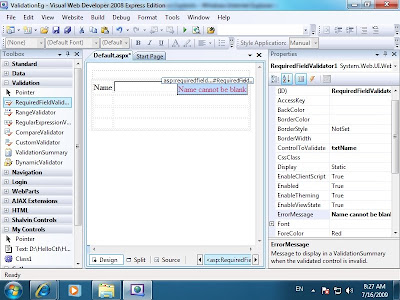
Result
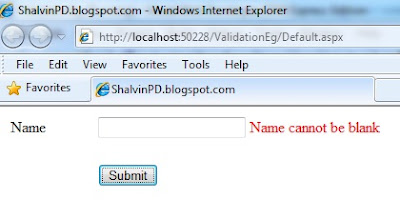
RequiredFieldValidator InitialValue
Suppose you want to display an additional message inside the textbox and that text shouldn't be treated as an input text. Then you can set the InitialValue of RequiredFieldValidator to the text that is displayed in the textbox.

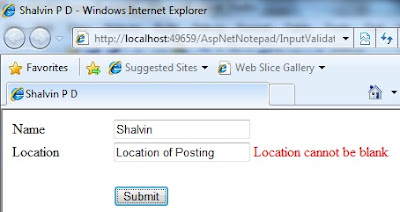
CompareValidator
CompareValidator can used for :
i. checking the type of contents in a control, for example whether the value entered is numeric.
ii. Check for equality of the contents in two controls, for example checking the text of Password and Reenter Password textboxes.
iii. Checking whether the values of two controls are greater than, lesss than, etc. for example wheter the value entered in Bid textbox is greater than the value in Price textbox.
OperatorCheck Property
i. Lets take up the first scenario. We want to check whether the value entered into a control is numeric.
As usual add a CompareValidator next to the control you want to validate. Set the ControlToValidate and ErrorMessage as in the case with RequiredFieldValidator. Set the Operator property to DataTypeCheck and Type to integer.
Now if the end user happens to enter a non numeric value he will receive an error message specified in the ErrorMessage property.
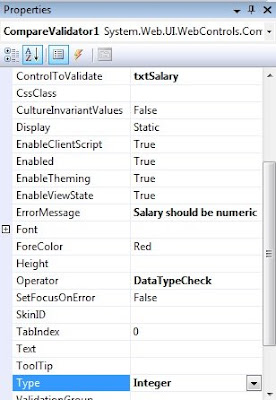
ControlToCompare Property
ii. Let's take up the scenario of checking the equality of values entered in two controls as in the case with Password and Reenter password textboxes.
Here set the ControlToValidate property to Re enter password textbox's id and ControlToCompare to the password textbox's id.
iii. In the case of third scenario where the Bid Amount should be greater than Price amount, set the ControlToValidate to Bid textbox's id and ControlToCompare to Price textbox's id. Next set the Operator to GreaterThan and Type to integer.
RangeValidator
RangeValidator can be used to check wheter the value entered into a control falls under a specific range. For example we can check wheter the age is between 35 and 60. In this case apart form ControlToValidate and ErrorMessge set the MinimumValue and MaximumValue property to 35 and 60 respectively.
ValidationSummary
Instead of displaying error message side by side the textbox, it can be displayed as summary. For this you can use ValidatiorSummary control. Place a ValidationSummar Control on the form having validation controls. Set the Text Property of Validation Controls to say *. All the controls that fail the validation text will display * as shown in the figure.
Asp .Net Ajax VII : AlwaysVisibleControlExtender
Friday, August 22, 2008
WPF Layout with Grid
WPF layout system helps in having fine grained control over the placing of controls.
Since I am more into the web domain I am most comfortable with the Grid which is the most versatile of the built in layout panels available in WPF.
Grid mimics the familiar html Table tags.
Here I am creating a Grid with 3 rows.
I am add two columns using ColumnDefinitions.
We can also specify the column number using Grid.Column.
<Window x:Class="WPFLayout.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPFLayout"
mc:Ignorable="d"
Title="Shalvin" Height="450" Width="800">
<Grid ShowGridLines="True">
<Grid.RowDefinitions>
<RowDefinition Height="50"/>
<RowDefinition Height="50"/>
<RowDefinition Height="50"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="150"/>
<ColumnDefinition Width="150"/>
<ColumnDefinition Width="150"/>
</Grid.ColumnDefinitions>
<Button>Shalvin.com</Button>
<Button Grid.Column="1">shalvinpd.blogspot.com</Button>
</Grid>
</Window>
Thursday, August 14, 2008
Wpf and Windows Forms Controls Interop
Though WPF is a Wonderful technology, at times programmers get frustrated with the lack of certain controls. One such control is the DataGrid or GridView. Silverlight 2 infact has a DataGrid, hope in the next release of WPF it will be as feature rich as Windows Forms.
It is very much possible to use Windows Forms controls in WPF.
Start out by dragging a WindowsFormsHost into WPF Windows.
Create an object of System.Windows.Forms..DataGrid and set the Child Property of WindowsFormsHost to the newly created DataGrid object. WindowsFormsHost can have only one child as is obvious form the Child Property.
System.Windows.Forms.DataGrid dg = new System.Windows.Forms.DataGrid();
private void Window_Loaded(object sender, RoutedEventArgs e)
{
windowsFormsHost1.Child = dg;
}
Now you can work with the DataGrid just like you work with it in Windows Forms.
Here is the complete code for connecting the DataGrid to a DataSet.
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms;
System.Windows.Forms.DataGrid dg = new System.Windows.Forms.DataGrid();
SqlConnection cnn;
SqlDataAdapter da;
DataSet ds = new DataSet();
private void Window_Loaded(object sender, RoutedEventArgs e)
{
windowsFormsHost1.Child = dg;
cnn = new SqlConnection(@"Integrated Security=sspi;Initial Catalog=Shalvin;Data source=.\sqlexpress");
cnn.Open();
da = new SqlDataAdapter("select * from Categories", cnn);
da.Fill(ds, "Cat");
dg.DataSource = ds.Tables["Cat"];
}
Sunday, July 6, 2008
.Net Code Access Security Introduction
CAS is the programatically means by which you secure the resouce of a system like file system, printer, registry, etc. in contrast to Role Base Security (RBS).
RequestOptional is used to grant permission to a resource.
RequestRefuse is used to revoke permission to a resource.
In following example I am going to restrict writing to C drive. Incase if user tries to write to C drive programatically he is receive an exception.
using System.Security.Permissions;
using System.Security;
using System.IO;
[assembly: FileIOPermissionAttribute(SecurityAction.RequestRefuse , Write="c:\\")]namespace WindowsFormsApplication1
{
public partial class Form1 : Form
{
StreamWriter sw;
private void button1_Click(object sender, EventArgs e)
{
sw = File.CreateText("c:\\Shalvin.txt");
sw.WriteLine("Hello");
sw.Close();
}
For a detailed coverage of Code Access Security refer http://www.codeproject.com/KB/security/UB_CAS_NET.aspx
Courtesy : Jeevan Baby, Indiaoptions
Thursday, June 26, 2008
My .Net Videos in Youtube/Google videos
Asp .Net Ajax Videos
Asp.Net Ajax II : TextBoxWatermarkExtender
Asp .Net Ajax III : ConfirmButtonExender
Asp .Net Ajax III : SliderExtender
Asp .Net Ajax V : MaskEditExtender
Asp .Net 2.0 Membership Vidoes
Asp.Net 2.0 Membership I : LoginStatus LoginName
ASP.Net 2.0 Membership II : LoginView Control
ASP.Net 2.0 Membership III - CreateUserWizard
ASP.Net 2.0 Membership IV Roles
aspnet_regsql : Adding Membership tables to Sql Server
WPF
WPF I : Button - Shalvin
Asp .net State Management
Asp .Net Query String
ASP.Net (VB.Net) Session Variable ArrayList
Asp .Net Profile
Asp .Net Controls
Asp.Net I : Introduction and Button Control
ASP.Net Controls - II : TextBox
Asp.Net III : ListBox Inline Page Mode IsPostBack
Asp.Net IV : Label AssociateControlId AccessKey
Implementing Date Selection Functionality using Multiple Combo Boxes
C#
String.Format
C# Error Handling - Shalvin P D
VB .Net
VB.Net 2008 Controls III :ListBox
VB .Net 2008 Controls V : NumericUpDown
VB .Net 2008 Controls VI : Simple Calculator
Google Videos
Creating Window Service in C#
C# (Windows Forms) MultiThreading
WPF Creating Class C#
Ado .Net
Introduction to Ado .Net
Ado .Net ExecuteScalar
Asp .Net
Displaying DataReader Contents in a RadioButtonList
Asp.Net (VB.Net) DropDownList DataTextField DataValueField
Asp.Net (VB.Net) Displaying DataReader contents in a RadioButtonList
ASP.Net DataList HyperLink with Wizard
FileUpload Control
Asp.Net C# DataSet GridView
ASP.Net (C#) Tracing
Asp .Net 2.0 Membership videos
ASP.Net Membership Authentication, Authorization and Role based security
Asp.Net 2.0 Membership V Denying Anonymous users
Asp.Net 2.0 Membership VIII Protecting a folder
C# Windows Forms
PrintDocument and PrintPreview Control
Gdi+
I : DrawString DrawLine
II : FillRectangle FillEllipse
III : Custom Pen
IV : HatchBrush
V: Color.FromArgb
VI :ScreenSaver : Random Class and Timer Control
VII : Working with Bitmaps
Gdi+ (ASP.Net) XII : Bitmap DrawString
Multithreading and Miscellaneous
Multithreading in Windows Forms
Code Access Security
Compression and Decompression in .Net
Win32 Api Visual Basic 6 : SwapMouseButton
Reflection in .Net : Displaying all properties, methods and events of a type
Xml
XmlDocument in VB .Net
Extracting xml from Sql Server tables
Sql Server
Sql Server 2005 : Creating Tables and Relationships (Sql)
Sql Server WHERE Clause and Stored Procedures
Web Service
Creating and consuming Web Service
Web Parts
Asp .Net Web Parts : Design Mode and Brose Mode